A Coding Guide to Build a Finance Analytics Tool for Extracting Yahoo Finance Data, Computing Financial Analysis, and Creating Custom PDF Reports
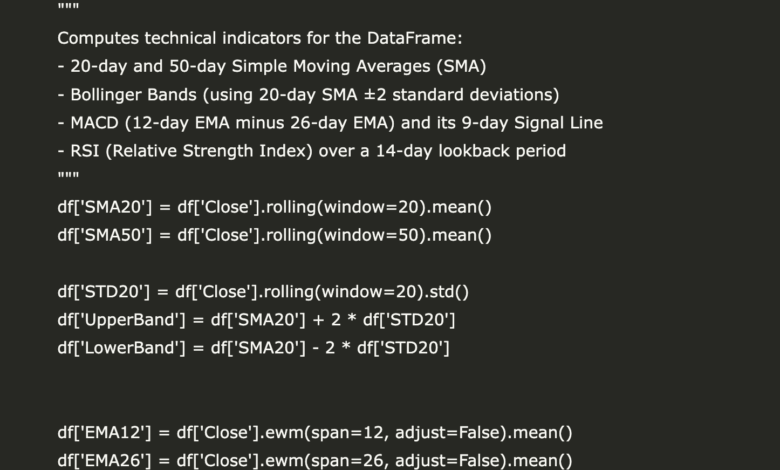
Extracting and analyzing stock data is a key to making enlightened decisions in the financial scene. This tutorial provides a comprehensive guide for building an integrated financial analysis tool and preparing reports in Python. We will learn to withdraw the historical market data from Yahoo Finance and calculate basic technical indicators such as simple moving averages, Bollegerer, MACD, and RSI. The guide walks by creating insightful perceptions and assembling them in the multi -page PDF reports. Whether you are a fan of data, financial analyst, or Python developer, who is looking to expand your tool group, this tutorial will shake you with skills that help convert raw market data into implemented visions.
import yfinance as yf
import pandas as pd
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.backends.backend_pdf import PdfPages
We import the basic Python libraries to analyze and visualize financial data. Here, Yfinance is used to bring in stock market data, pandas for data processing, MATPLOTLIB and Numby to create and process numerical charts, and PDFPages to collect multiple plots in one PDF report.
def compute_indicators(df):
"""
Computes technical indicators for the DataFrame:
- 20-day and 50-day Simple Moving Averages (SMA)
- Bollinger Bands (using 20-day SMA ±2 standard deviations)
- MACD (12-day EMA minus 26-day EMA) and its 9-day Signal Line
- RSI (Relative Strength Index) over a 14-day lookback period
"""
df['SMA20'] = df['Close'].rolling(window=20).mean()
df['SMA50'] = df['Close'].rolling(window=50).mean()
df['STD20'] = df['Close'].rolling(window=20).std()
df['UpperBand'] = df['SMA20'] + 2 * df['STD20']
df['LowerBand'] = df['SMA20'] - 2 * df['STD20']
df['EMA12'] = df['Close'].ewm(span=12, adjust=False).mean()
df['EMA26'] = df['Close'].ewm(span=26, adjust=False).mean()
df['MACD'] = df['EMA12'] - df['EMA26']
df['Signal'] = df['MACD'].ewm(span=9, adjust=False).mean()
delta = df['Close'].diff()
gain = delta.copy()
loss = delta.copy()
gain[gain < 0] = 0
loss[loss > 0] = 0
loss = loss.abs()
avg_gain = gain.rolling(window=14).mean()
avg_loss = loss.rolling(window=14).mean()
rs = avg_gain / avg_loss
df['RSI'] = 100 - (100 / (1 + rs))
return df
This function calculates the main technical indicators – including SMAS, Bollenger, MACD, and RSI – for the stock price data in Dataframe input. It updates Dataframe with additional columns for each indicator, allowing in -depth technical analysis to perform historical stocks.
def create_cover_page(pdf):
"""
Creates and saves a cover page into the PDF report.
"""
fig = plt.figure(figsize=(11.69, 8.27))
plt.axis('off')
plt.text(0.5, 0.7, "Financial Analysis Report", fontsize=24, ha="center")
plt.text(0.5, 0.62, "Analysis of 5 Stocks from Yahoo Finance", fontsize=16, ha="center")
plt.text(0.5, 0.5, "Includes Technical Indicators: SMA, Bollinger Bands, MACD, RSI", fontsize=12, ha="center")
plt.text(0.5, 0.4, "Generated with Python and matplotlib", fontsize=10, ha="center")
pdf.savefig(fig)
plt.close(fig)
This function creates a visual cover page using Matplotlib and adds it as a first page of the PDF report via the submitted PDFPAGAS object. Then the number is closed to free resources.
def plot_price_chart(ticker, df):
"""
Generates a price chart with SMA and Bollinger Bands for a given ticker.
"""
fig, ax = plt.subplots(figsize=(14, 7))
ax.plot(df.index, df['Close'], label="Close Price", linewidth=1.5)
ax.plot(df.index, df['SMA20'], label="SMA (20)", linewidth=1.2)
ax.plot(df.index, df['SMA50'], label="SMA (50)", linewidth=1.2)
ax.plot(df.index, df['UpperBand'], label="Upper Bollinger Band", linestyle="--")
ax.plot(df.index, df['LowerBand'], label="Lower Bollinger Band", linestyle="--")
ax.fill_between(df.index, df['LowerBand'], df['UpperBand'], color="lightgray", alpha=0.3)
ax.set_title(f'{ticker}: Price & Moving Averages with Bollinger Bands')
ax.set_xlabel('Date')
ax.set_ylabel('Price')
ax.legend()
ax.grid(True)
return fig
This function is generated as a comprehensive plan for stock prices for a certain bunch that includes the close price, SMAS for 20 days and 50 days, and bollygerer gangs. It restores the Matplotlib character that can be saved or processed in a PDF report.
def plot_macd_chart(ticker, df):
"""
Generates a MACD plot for the given ticker.
"""
fig, ax = plt.subplots(figsize=(14, 5))
ax.plot(df.index, df['MACD'], label="MACD", linewidth=1.5)
ax.plot(df.index, df['Signal'], label="Signal Line", linewidth=1.5)
ax.set_title(f'{ticker}: MACD')
ax.set_xlabel('Date')
ax.set_ylabel('MACD')
ax.legend()
ax.grid(True)
return fig
This job creates a MACD scheme for the specified amount by drawing MACD and its signal line over time. It restores the Matplotlib character that can be combined into a larger report than PDF or independently displayed.
def plot_rsi_chart(ticker, df):
"""
Generates an RSI plot for the given ticker.
"""
fig, ax = plt.subplots(figsize=(14, 5))
ax.plot(df.index, df['RSI'], label="RSI", linewidth=1.5)
ax.axhline(70, color="red", linestyle="--", linewidth=1, label="Overbought (70)")
ax.axhline(30, color="green", linestyle="--", linewidth=1, label="Oversold (30)")
ax.set_title(f'{ticker}: RSI')
ax.set_xlabel('Date')
ax.set_ylabel('RSI')
ax.legend()
ax.grid(True)
return fig
This function creates the RSI chart for a specific stock scheme, planning the RSI values along with horizontal reference lines on peak levels (70) and increasing the sale (30). It restores the Matplotlib character that can be combined into the final financial analysis report.
def main():
tickers = []
for i in range(5):
ticker = input(f"Enter ticker #{i+1}: ").upper().strip()
tickers.append(ticker)
pdf_filename = "financial_report.pdf"
with PdfPages(pdf_filename) as pdf:
create_cover_page(pdf)
for ticker in tickers:
print(f"Downloading data for {ticker} from Yahoo Finance...")
df = yf.download(ticker, period='1y')
if df.empty:
print(f"No data found for {ticker}. Skipping to the next ticker.")
continue
df = compute_indicators(df)
fig_price = plot_price_chart(ticker, df)
pdf.savefig(fig_price)
plt.close(fig_price)
fig_macd = plot_macd_chart(ticker, df)
pdf.savefig(fig_macd)
plt.close(fig_macd)
fig_rsi = plot_rsi_chart(ticker, df)
pdf.savefig(fig_rsi)
plt.close(fig_rsi)
print(f"PDF report generated and saved as '{pdf_filename}'.")
Here, this main function of the user is required to enter five stock indicators, one -year data download for Yahoo Financing, the main technical indicators, the corresponding price generation, MACD, and RSI. Then it collects all the plans in the multi -page PDF report called “Financial_report.PDF” and prints a confirmation message once the report is saved.
if __name__ == "__main__":
main()
Finally, this mass is achieved whether the text program is implemented directly instead of importing it as a stereotype. If so, it calls the main function ().
In conclusion, we have shown a way to automate the financial analysis using Python. You have learned how to extract valuable data, calculate the main technical indicators, and create comprehensive visual reports in the multi -page PDF format. This integrated approach simplifies the analysis process and provides a strong way to visualize market trends and monitor stock performance. While allocating this framework and expanding this framework, you can continue to enhance your analytical abilities and make more enlightened financial decisions.
Here is Clap notebook. Also, do not forget to follow us twitter And join us Telegram channel and LinkedIn GrOup. Don’t forget to join 85k+ ml subreddit.
🔥 [Register Now] The virtual Minicon Conference on Agency AI: Free Registration + attendance Certificate + 4 hours short (May 21, 9 am- Pacific time)
SANA Hassan, consultant coach at Marktechpost and a double -class student in Iit Madras, is excited to apply technology and AI to face challenges in the real world. With great interest in solving practical problems, it brings a new perspective to the intersection of artificial intelligence and real life solutions.
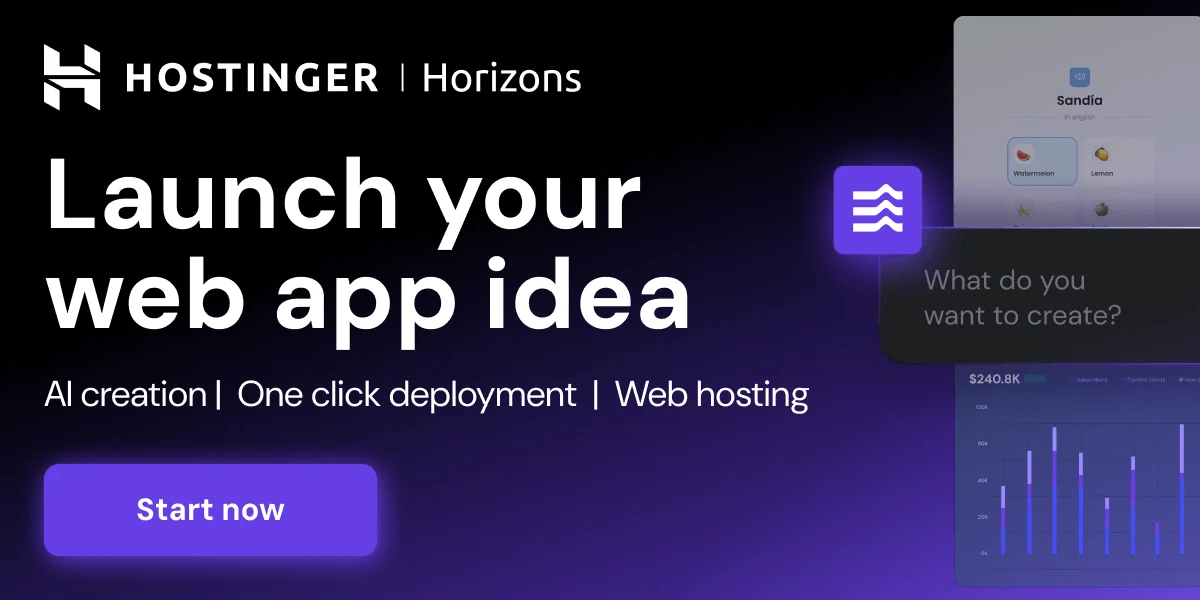
Don’t miss more hot News like this! Click here to discover the latest in AI news!
2025-04-15 06:27:00